Swap All Values on Click
Function
UI & UX
</ head>
</ body>
<!-- SWAP VALUES ON CLICK -->
<script>
function setupSwitcher({
/* Click Trigger Class */
swapTrigger = "switcher",
/* Essentials */
swapText = "swap-text",
swapDisplay = "swap-display",
swapClass = "swap-class",
swapUrl = "swap-url",
/* Toggle and Add Attributes */
swapToggleAttribute = "swap-toggle-attribute",
swapAddAttribute = "swap-add-attribute",
swapAddAttributeValue = "swap-add-attribute-value",
/* Replace Attribute Value */
swapAttributeTarget = "swap-attribute-target",
swapTargetValue = "swap-target-value"
} = {}) {
const swapAll = () => {
// SWAP TEXT
document.querySelectorAll(`[${swapText}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapText);
const currentValue = swapDomElement.innerText;
swapDomElement.innerText = nextValue;
swapDomElement.setAttribute(swapText, currentValue);
});
// SWAP DISPLAY
document.querySelectorAll(`[${swapDisplay}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapDisplay);
const currentValue = swapDomElement.style.display;
swapDomElement.style.display = nextValue;
swapDomElement.setAttribute(swapDisplay, currentValue);
});
// SWAP CLASS
document.querySelectorAll(`[${swapClass}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapClass);
swapDomElement.classList.toggle(nextValue);
});
// SWAP URL
document.querySelectorAll(`[${swapUrl}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapUrl);
const currentValue = swapDomElement.href;
swapDomElement.href = nextValue;
swapDomElement.setAttribute(swapUrl, currentValue);
});
// TOGGLE ATTRIBUTE
document.querySelectorAll(`[${swapToggleAttribute}]`).forEach((swapDomElement) => {
const toggleAttribute = swapDomElement.getAttribute(
swapToggleAttribute
);
swapDomElement.toggleAttribute(toggleAttribute);
});
// ADD ATTRIBUTE
document.querySelectorAll(`[${swapAddAttribute}]`).forEach((swapDomElement) => {
const newAttribute = swapDomElement.getAttribute(swapAddAttribute);
const newAttributeValue = swapDomElement.getAttribute(
swapAddAttributeValue
);
if (swapDomElement.hasAttribute(newAttribute)) {
swapDomElement.removeAttribute(newAttribute);
} else {
swapDomElement.setAttribute(newAttribute, newAttributeValue);
}
});
// REPLACE ATTRIBUTE VALUE
document.querySelectorAll(`[${swapAttributeTarget}]`).forEach((swapDomElement) => {
const attributeTarget = swapDomElement.getAttribute(
swapAttributeTarget
);
let attributeValue = swapDomElement.getAttribute(attributeTarget);
let newValue = swapDomElement.getAttribute(swapTargetValue);
swapDomElement.setAttribute(attributeTarget, newValue);
swapDomElement.setAttribute(swapTargetValue, attributeValue);
});
};
document.querySelectorAll(`.${swapTrigger}`).forEach((switcherDomElement) => {
switcherDomElement.addEventListener("click", swapAll);
});
}
setupSwitcher();
</script>
HTML/Embed
Only tabs with a " " have code.
<!-- SWAP VALUES ON CLICK -->
<script>
function setupSwitcher({
/* Click Trigger Class */
swapTrigger = "switcher",
/* Essentials */
swapText = "swap-text",
swapDisplay = "swap-display",
swapClass = "swap-class",
swapUrl = "swap-url",
/* Toggle and Add Attributes */
swapToggleAttribute = "swap-toggle-attribute",
swapAddAttribute = "swap-add-attribute",
swapAddAttributeValue = "swap-add-attribute-value",
/* Replace Attribute Value */
swapAttributeTarget = "swap-attribute-target",
swapTargetValue = "swap-target-value"
} = {}) {
const swapAll = () => {
// SWAP TEXT
document.querySelectorAll(`[${swapText}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapText);
const currentValue = swapDomElement.innerText;
swapDomElement.innerText = nextValue;
swapDomElement.setAttribute(swapText, currentValue);
});
// SWAP DISPLAY
document.querySelectorAll(`[${swapDisplay}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapDisplay);
const currentValue = swapDomElement.style.display;
swapDomElement.style.display = nextValue;
swapDomElement.setAttribute(swapDisplay, currentValue);
});
// SWAP CLASS
document.querySelectorAll(`[${swapClass}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapClass);
swapDomElement.classList.toggle(nextValue);
});
// SWAP URL
document.querySelectorAll(`[${swapUrl}]`).forEach((swapDomElement) => {
const nextValue = swapDomElement.getAttribute(swapUrl);
const currentValue = swapDomElement.href;
swapDomElement.href = nextValue;
swapDomElement.setAttribute(swapUrl, currentValue);
});
// TOGGLE ATTRIBUTE
document.querySelectorAll(`[${swapToggleAttribute}]`).forEach((swapDomElement) => {
const toggleAttribute = swapDomElement.getAttribute(
swapToggleAttribute
);
swapDomElement.toggleAttribute(toggleAttribute);
});
// ADD ATTRIBUTE
document.querySelectorAll(`[${swapAddAttribute}]`).forEach((swapDomElement) => {
const newAttribute = swapDomElement.getAttribute(swapAddAttribute);
const newAttributeValue = swapDomElement.getAttribute(
swapAddAttributeValue
);
if (swapDomElement.hasAttribute(newAttribute)) {
swapDomElement.removeAttribute(newAttribute);
} else {
swapDomElement.setAttribute(newAttribute, newAttributeValue);
}
});
// REPLACE ATTRIBUTE VALUE
document.querySelectorAll(`[${swapAttributeTarget}]`).forEach((swapDomElement) => {
const attributeTarget = swapDomElement.getAttribute(
swapAttributeTarget
);
let attributeValue = swapDomElement.getAttribute(attributeTarget);
let newValue = swapDomElement.getAttribute(swapTargetValue);
swapDomElement.setAttribute(attributeTarget, newValue);
swapDomElement.setAttribute(swapTargetValue, attributeValue);
});
};
document.querySelectorAll(`.${swapTrigger}`).forEach((switcherDomElement) => {
switcherDomElement.addEventListener("click", swapAll);
});
}
setupSwitcher();
</script>
Only tabs with a " " have code.
Only tabs with a " " have code.
Only tabs with a " " have code.
If you have already pasted this code into your project then you can skip this. If you haven't, and it's your first time using CodeCrumbs, then copy this code and navigate to your sites global settings > Custom Code tab > paste it into the <head> (first custom code block). It just needs to exist once.
How to use:
Unlock more with PRO
Want to learn how to use this Crumb? Unlock detailed documentation, video tutorials, comments & support!
Upgrade to Pro
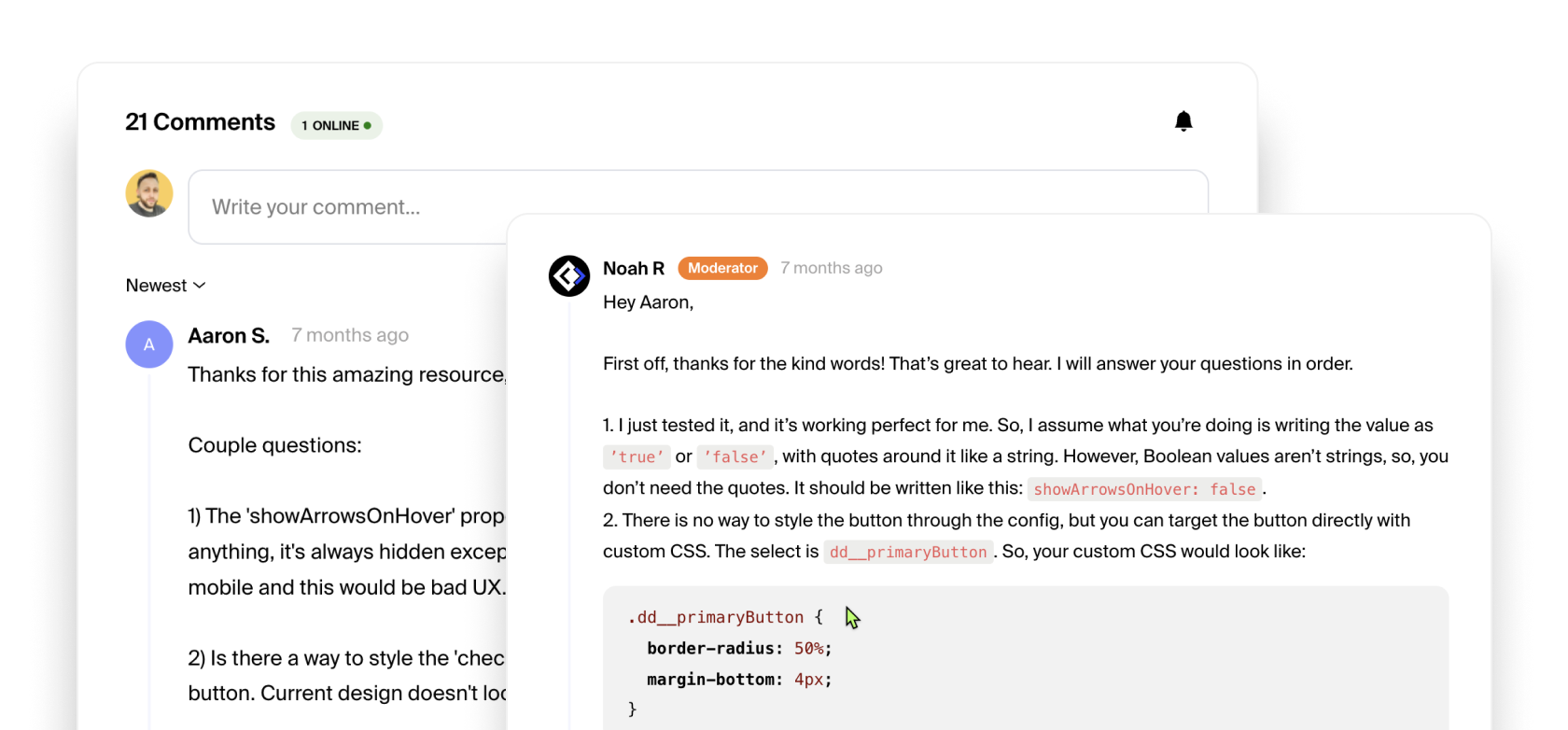
Unlock more with PRO
Want to learn how to use this Crumb? Unlock detailed documentation, video tutorials, comments & support!
Upgrade to Pro
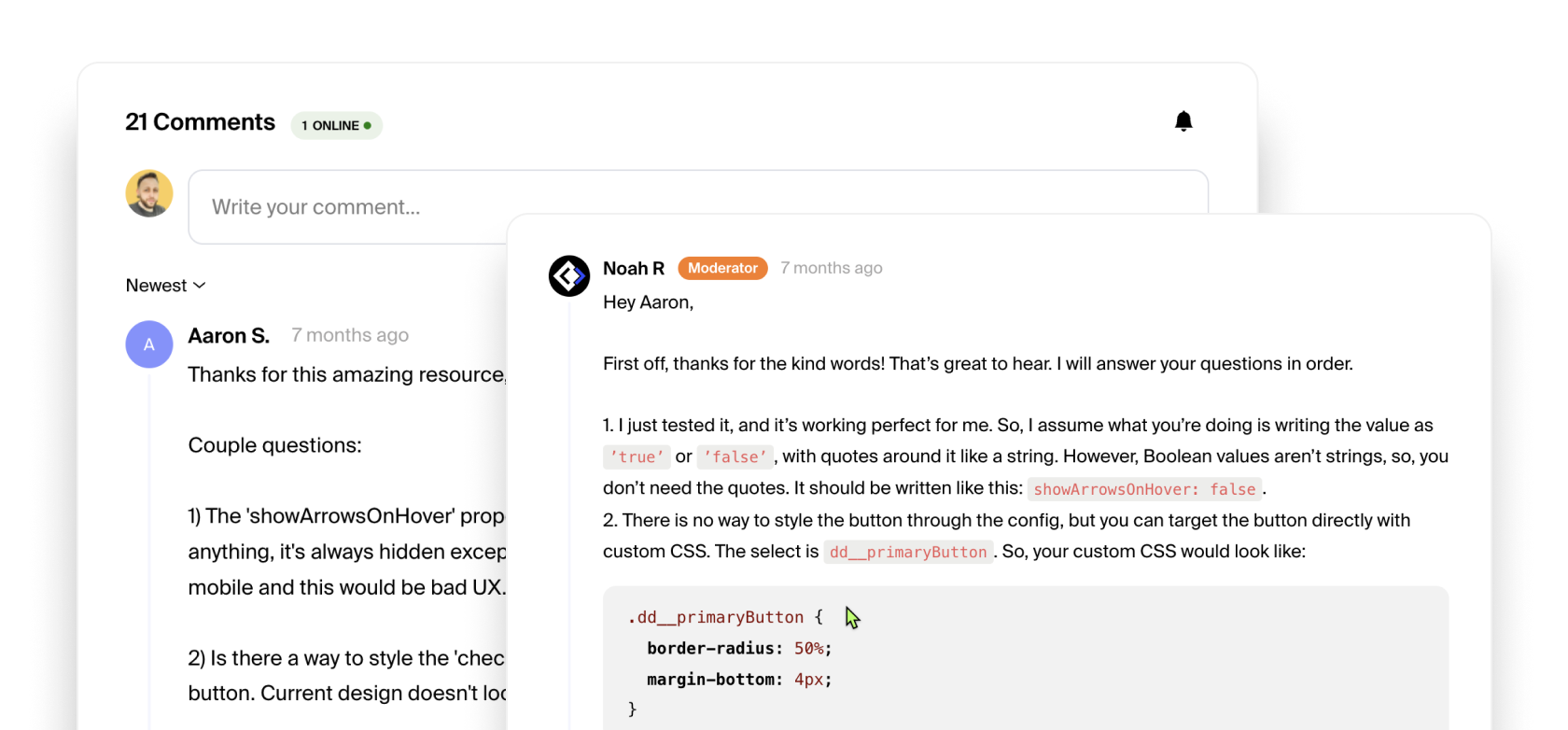
Tutorial Coming Soon!
Check Browser Support
Interactive Table
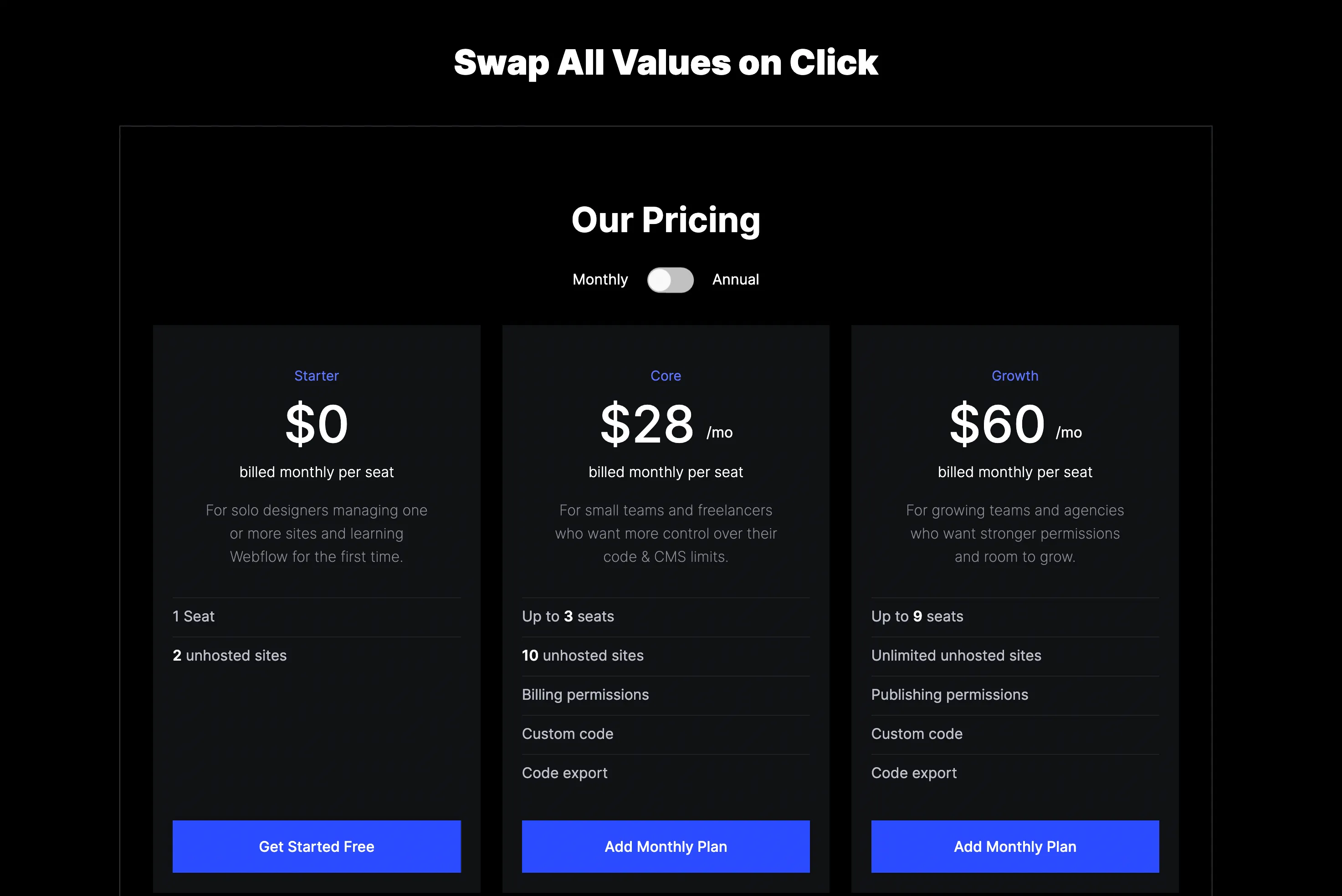
Contributor
CodeCrumbs Team
Short Description:
One click trigger to change & swap text, urls, attributes, display properties and more.
Extra Info:
No extra info.
Clone Project
Documentation
Author:
CodeCrumbs
Status:
Deprecated
New
Updated
This crumb is no longer being supported.
Latest Version:
More related crumbs..
Want to contribute to the community?
Tell us what code you're working with. We would love to see it and possibly add it to the library.